Hello friends, in this article I will write about How to upgrade from ASP.NET Core 3.1 to .NET 6.0.
To demonstrate this, I have developed a basic CRUD application in .NET Core 3.1 using Visual Studio 2022.
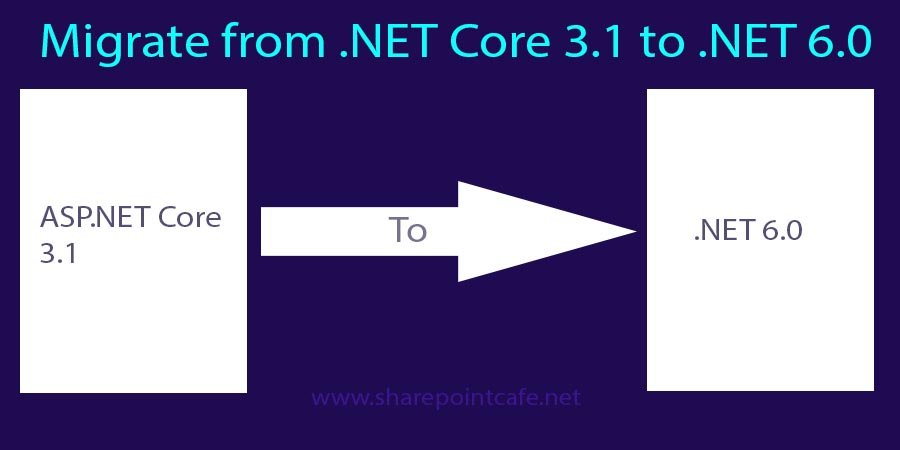
Pre-requisites
To upgrade from ASP.NET Core 3.1 to .NET 6, you should have the below tools, SDK, IDE –
- Visual Studio 2022
- .NET 6 SDK
- SQL Server 2017 and above
- Microsoft.EntityFrameworkCore.SqlServer 5.0.0
Introduction to .NET Core 3.1 based Project
I have a very basic application to perform CRUD operations. The application was developed in Visual Studio 2022 and the base framework is .NET Core 3.1.
Read this also - Migrate from ASP.NET Core 2.2 to ASP.NET Core 3.1
Below is the Controller Class Code snippet.
using BasicAPI.Models;
using BasicAPI.Service;
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
// For more information on enabling Web API for empty projects, visit https://go.microsoft.com/fwlink/?LinkID=397860
namespace BasicAPI.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ProductsController : ControllerBase
{
private readonly IProductService _productService;
public ProductsController (IProductService productService)
{
_productService = productService;
}
// GET: api/<ProductsController>
[HttpGet]
[Route("ProductList")]
public IEnumerable<Product> GetProductList()
{
return _productService.GetProductList();
}
// GET api/<ProductsController>/5
[Route("ProductDetails")]
public Product Get(int id)
{
return _productService.GetProductById(id);
}
// POST api/<ProductsController>
[HttpPost]
[Route("AddProduct")]
public IActionResult AddProducts(Product product)
{
_productService.AddProductDetails(product);
return Ok();
}
// PUT api/<ProductsController>/5
[HttpPut("{id}")]
public void Put(int id, [FromBody] string value)
{
}
// DELETE api/<ProductsController>/5
[HttpDelete("{id}")]
public void Delete(int id)
{
}
}
}
Model Class –
Below is the code snippet of Model class. In my case Model name is Product.
namespace BasicAPI.Models
{
public class Product
{
public int Id { get; set; }
public string ProductCode { get; set; }
public string ProductName { get; set; }
public int Category { get; set; }
public decimal Cost { get; set; }
public decimal Stock { get; set; }
}
}
Below is the DB Context file.
using BasicAPI.Models;
using Microsoft.EntityFrameworkCore;
namespace BasicAPI.DataContext
{
public class ProductContext:DbContext
{
public ProductContext(DbContextOptions<ProductContext> options) : base(options)
{
}
public DbSet<Product> Products { get; set; }
}
}
Code snippet is of service interface.
using BasicAPI.Models;
using System.Collections.Generic;
namespace BasicAPI.Service
{
public interface IProductService
{
Product AddProductDetails(Product product);
List<Product> GetProductList();
void UpdateProduct(Product product);
void DeleteProduct(int Id);
Product GetProductById(int Id);
}
}
Below is the Service Implementation class.
using BasicAPI.DataContext;
using BasicAPI.Models;
using System.Collections.Generic;
using System.Linq;
namespace BasicAPI.Service
{
public class ProductService : IProductService
{
public ProductContext _productContext;
public ProductService(ProductContext productContext)
{
_productContext = productContext;
}
public Product AddProductDetails(Product product)
{
_productContext.Products.Add(product);
_productContext.SaveChanges();
return product;
}
public void DeleteProduct(int Id)
{
throw new System.NotImplementedException();
}
public Product GetProductById(int Id)
{
return _productContext.Products.FirstOrDefault(p => p.Id == Id);
}
public List<Product> GetProductList()
{
return _productContext.Products.ToList();
}
public void UpdateProduct(Product product)
{
throw new System.NotImplementedException();
}
}
}
Database Object –
Create table script.
/****** Object: Table [dbo].[Products] Script Date: 30-01-2022 19:46:53 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE TABLE [dbo].[Products](
[Id] [int] IDENTITY(1,1) NOT NULL,
[ProductCode] [nvarchar](50) NULL,
[ProductName] [nvarchar](50) NULL,
[Category] [int] NULL,
[Cost] [decimal](18, 2) NULL,
[Stock] [numeric](18, 0) NULL,
CONSTRAINT [PK_Products] PRIMARY KEY CLUSTERED
(
[Id] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON, OPTIMIZE_FOR_SEQUENTIAL_KEY = OFF) ON [PRIMARY]
) ON [PRIMARY]
GO
Steps to Migrate or Upgrade from ASP.NET Core 3.1 to .NET 6.0
Follow the below steps to migrate your current ASP.NET Core 3.1 based application to .NET 6.0.
Step 1 – Open your current project in Visual Studio 2022. Right-click on the Project name in solution explorer and select Properties.
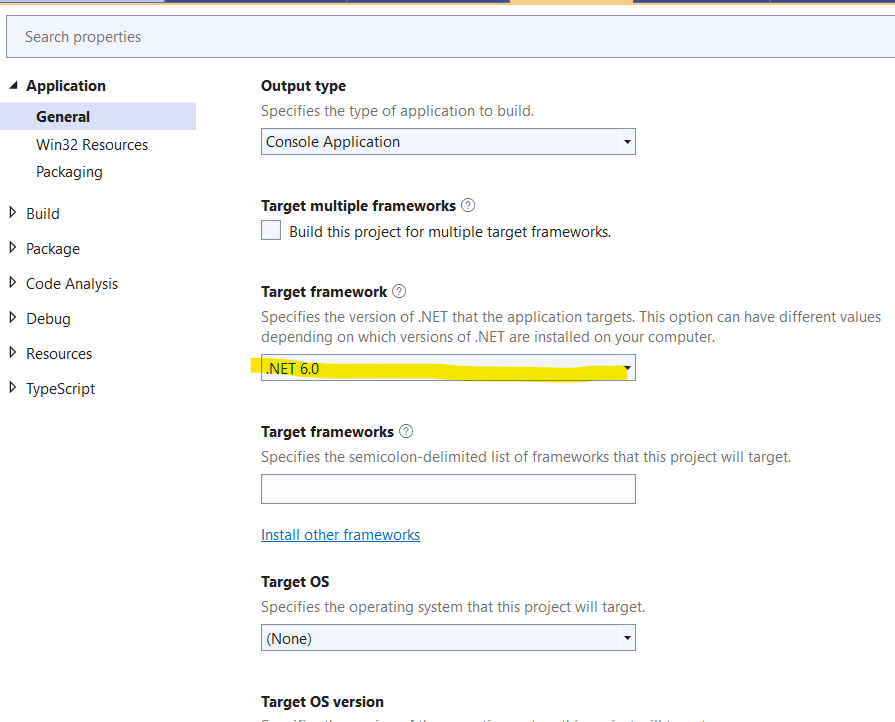
Step 2 – Change the Target framework to .NET 6.0 as shown in the above image.
Your base framework is now changed. To check this, right-click on the project name in solution explorer and click Edit Project File.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net6.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="5.0.0" />
<PackageReference Include="Newtonsoft.Json" Version="13.0.1" />
</ItemGroup>
</Project>
Step 3 – We need to upgrade the packages that have been used to run the application.
While migrating from ASP.NET Core 3.1 to .NET 6.0 it is very important to upgrade the nuget packages used in the current project.
In my case, I have used only one package – Microsoft.EntityFrameworkCore.SqlServer 5.0.0 as this is a very basic API project.
To make it compatible to .NET 6, upgrade this via nuget Package Manager.

Step 4 – Right-click on the project name in solution explorer and select Build. Your application should build successfully.
Step 5 – Remove bin and obj folders and clear cache. You should delete the bin and obj folders. Also, run dotnet nuget locals –clear all to clear the NuGet package cache.
Optional steps
Now, the below steps are completely optional. If you want to use it you can use else leave it.
Step 6 – In .NET 6 there is no startup.cs file and the code snippet found in startup.cs is merged in Program.cs file. So, if you will create a new .NET 6 project in Visual Studio 2022 then you may see only Program.cs file, but in up-gradation case it retains startup.cs file.
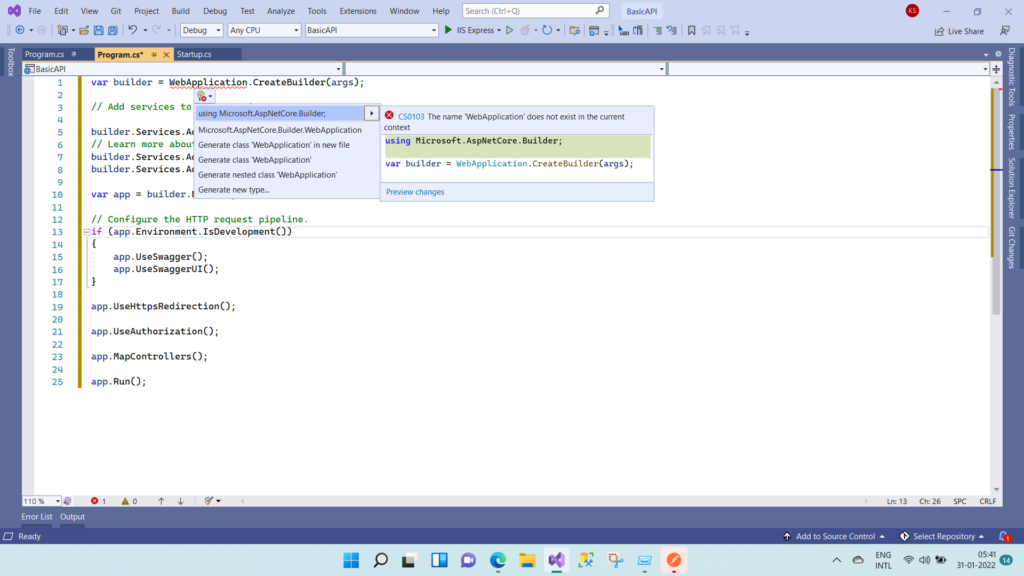
Step 7 – Add Swashbuckle.AspNetCore NuGet package.
You need to add Swashbuckle.AspNetCore package in case you want to use it.

Step 8 – Add the Connection string in Program.cs file –
Below is the code snippet to define the connection string.
builder.Services.AddDbContext<ProductContext>(options =>
{
options.UseSqlServer(builder.Configuration.GetConnectionString("DefaultConnectionString"));
});
Step 9 – Remove existing code from Program.cs file and copy the below code snippet in Program.cs file.
You may notice that code written in Configure() method in .NET Core 3.1 is now merged in Program.cs file.
using BasicAPI.DataContext;
using BasicAPI.Service;
using Microsoft.AspNetCore.Builder;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
builder.Services.AddDbContext<ProductContext>(options =>
{
options.UseSqlServer(builder.Configuration.GetConnectionString("DefaultConnectionString"));
});
builder.Services.AddScoped<IProductService, ProductService>();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
Step 10 – Now, run your application and check the output.
Summary –
We have migrated a very basic .NET Core 3.1-based project to .NET 6 version. Migration tasks depend on how many services, features, and libraries you have used in your older version.
The more libraries and features mean the more up-gradation or migration steps.
To demonstrate this article I have used a very basic CRUD operation with Entity Framework which I did very easily.
Note: Migration from ASP.NET Core 3.1 to .NET 6.0 may vary from project to project.
You may refer to the below link for more details – Migrate from ASP.NET Core 3.1 to 6.0 | Microsoft Docs
Hope you like this article and it might be useful for you.