In this article, I will write down the process which I used to Migrate from ASP.NET Core 2.2 project to .NET Core 3.1.
Read my previous articles which are related to the migration process –
.NET Core 3.1 API returns empty JSON objects How to check the C# version in Visual Studio 2022?
My Current Project is ASP.NET Core Web API and developed in Visual Studio 2017, the framework is ASP.NET Core 2.2. To migrate the project to .NET Core 3.1 we need Visual Studio 2019 or a later version.
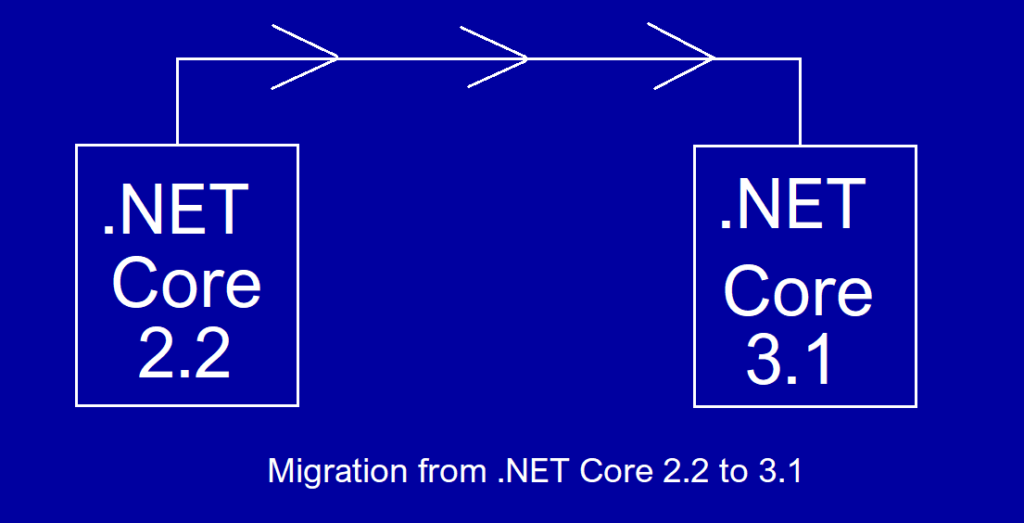
Prerequisites
- Visual Studio 2019
- .NET Core 3.1 SDK
Steps to Migrate from ASP.NET Core 2.2 project to .NET Core 3.1
Software migration is always a risky and little tedious task as there could be several impacts on the project. But in this article, I will write about step by step process which will help you to migrate your current .NET Core 2.2 project to .NET Core 3.1
Please follow the below steps to complete the migration successfully.
Step 1 – Open your project/ application in Visual Studio 2019
Step 2 – Right-click on your project name in solution explorer and select edit Project file.
Change the target framework as per the below code snippet.
<TargetFramework>netcoreapp3.1</TargetFramework>
Step 3 – Open Startup.cs file and follow the below changes.
Changes in ConfigureServices() method
Remove below line from ConfigureServices method.
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
And add this line in ConfigureServices() method-
services.AddControllers();
Changes in Configure() method
In Configure() method parameter, replace IHostingEnvironment with IWebHostEnvironment.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, ILoggerManager logger)
You need to add this namespace – Microsoft.Extensions.Hosting;
Comment app.UseMvc() in Configure() method and add below code snippet –
app.UseHttpsRedirection();
app.UseRouting();
app.UseCors();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
Step 4 – Open Program.cs file
IWebHostBuilder interface is now IHostBuilder.
Old Code snippet-
public static IWebHostBuilder CreateWebHostBuilder(string[] args) =>
WebHost.CreateDefaultBuilder(args)
.UseStartup<Startup>();
New code snippet –
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
Complete Program.cs file –
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
}
Step 5 – Install Microsoft.EntityFrameworkCore.SqlServer
If you have used EntityFrameworkCore then you need to install this package. Initially, I installed 3.1 version of this package but identified huge changes because of the way it was written in .NET Core 2.2.
This is because FromSql() method is now FromSqlRaw(). Also, the new function requires to add AsEnumerable right after FromSqlRaw() method.
I have installed Microsoft.EntityFrameworkCore.SqlServer version 2.1.0 to avoid any changes in data access layer files.
Step 6 – nuGet Package updates
If you have used any library which needs to be updated then please do that with nuGet Package manager.
For example – Newtonsoft.json.
Issues need to resolve post Migrating to .NET Core 3.1
After completing all the steps mentioned above, the migration process was done.
Although, there could be some random issues that can occur for some of the users.
Issue #1 – If you are using entity framework core and upgraded to Entity Framework Core 3.1 then FromSql() method need to rename as FromSqlRaw(). Starting with EF Core 3.0, use FromSqlRaw, ExecuteSqlRaw, and ExecuteSqlRawAsync to create a parameterized query where the parameters are passed separately from the query string.
Reference - Breaking changes included in EF Core 3.x
If you are using the stored procedure, then you can add AsEnumerable/AsAsyncEnumerable right after the FromSqlRaw method.
context.Products.FromSqlRaw("[dbo].[GetList]").AsEnumerable().FirstOrDefault();
In my case, I installed Microsoft.EntityFrameworkCore.SqlServer (Version 2.1.0) to avoid any changes in the data access layer.
If you are comfortable updating the data access layer files then you may install Microsoft.EntityFrameworkCore.SqlServer (Version 3.1).
Issue #2 – This issue is quite surprising for me. There is neither compile-time error nor run time error. It just gives an empty JSON object as shown below.
[
[
[
[]
],
[
[]
]
]
]
Below is the code snippet of the action method in one of the controller classes –
[HttpPost]
[Route("TestAPI")]
public IActionResult TestAPI()
{
string jsonData = @"[{ 'Country': 'India', 'City': 'New Delhi'}]";
return Ok(JArray.Parse(jsonData));
}
To resolve this, change the return statement as shown below.
return Content(jsonData.ToString(), "application/json", Encoding.UTF8);
Finally, you can see the output as expected from your service endpoint.
Please do proper testing and follow all test cases or requirements before moving the upgraded code to the production environment.
Hope you like this article on “How to Migrate from ASP.NET Core 2.2 project to .NET Core 3.1?”
If you have any feedback or suggestion then you are most welcome and please write them down below in the comment box.