In this Azure article, we will discuss How to Extract Text from an Image Using Azure Cognitive Services, we will also see a few other topics like Azure Cognitive Services to Read Text from Images with Hand-written text.
We will perform the below steps to demonstrate the functionality.
- We will create an Azure AI Computer Vision resource in the Azure Portal to generate the Key and endpoint.
- Next, we will create a Console App in .NET Core using Visual Studio 2022.
Azure Cognitive Service to extract text from images
Microsoft Azure offers Azure AI Computer Vision service that processes images and provides data using algorithms. Let’s create a Computer Vision resource in Azure Portal and write C# Code to read text from an image.
The Read API can extract text from photos using AI techniques and return it as structured strings.
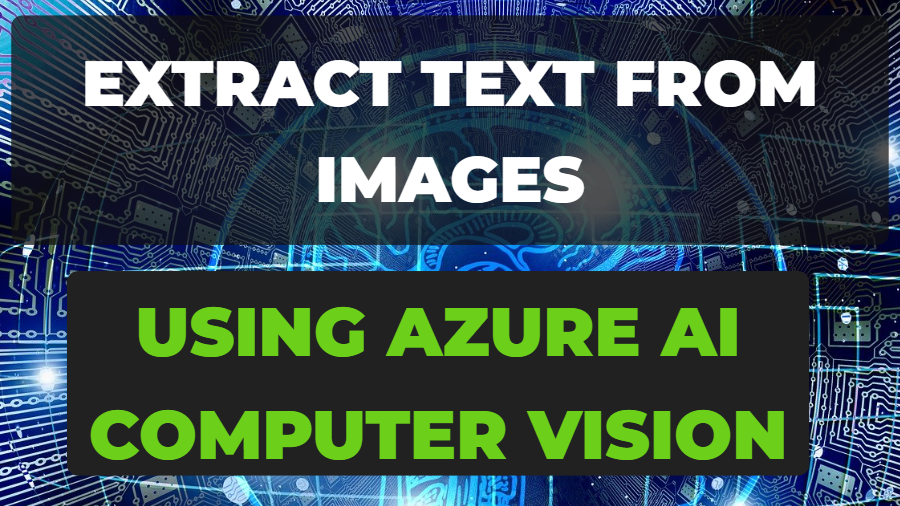
Through AI enrichment, Azure Cognitive Search gives you several options for creating and extracting searchable text from images, including:
- OCR
- Image Analysis
What is Azure Cognitive Service?
Microsoft Cognitive Services, formerly Project Oxford, enables us to create intelligent apps by using their libraries. All of the APIs are built on machine learning APIs and it allows programmers to quickly incorporate intelligent features such as emotion and video detection, face, speech, and language understanding into their apps.
For more information about Azure Cognitive Service, please read this article.
What is OCR?
The full form of OCR is Optical Character Recognition. Text extraction or text recognition are other names for optical character recognition (OCR). You may extract text from photos, such as posters, and business cards using machine-learning-based OCR algorithms.
OCR Intelligent Engine
The Read OCR engine from Microsoft is made up of numerous sophisticated machine-learning models that support various languages. It can extract text from both printed and handwritten documents, even when the languages and writing styles are intermingled.
Prerequisites to implement Azure Cognitive Services
- An Azure subscription.
- The Visual Studio IDE with the current version of .NET Core.
- An Azure AI Vision resource. We will create this later in this article.
- The key and endpoint from the compute vision resource.
How Azure AI Computer Vision Performs Text Extraction?
In this section of the article, we will create Azure AI Computer Vision resource which is part of Azure Cognitive Service.
First, log in to your Azure Portal. If you do not have an Azure subscription, Click here to proceed.
Follow the below steps to create Azure Computer Vision and proceed further.
Post successful Login to your Azure Portal, and click on More Services.

Then select AI + machine learning from the left side panel, as shown in the below screenshot.
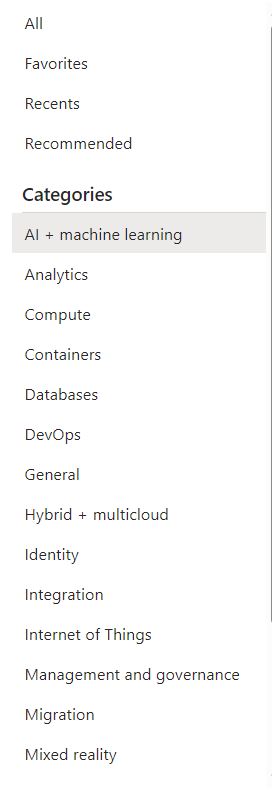
Select Computer vision from the available list.

Click on Create and fill in the required fields.

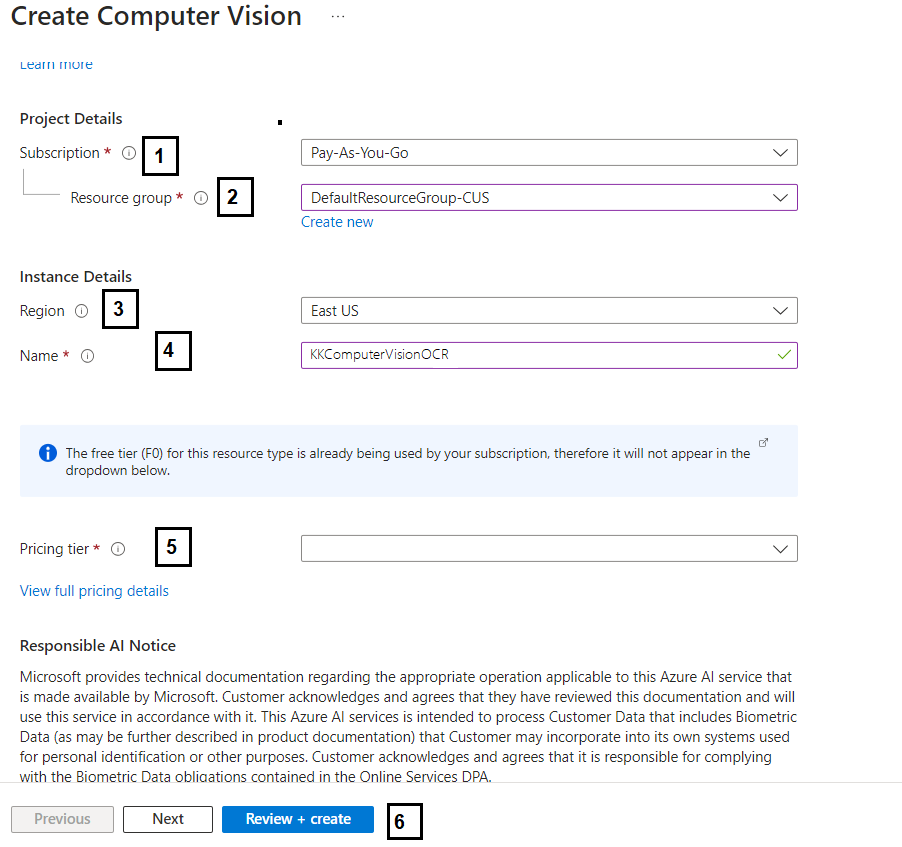
You may select the Free pricing tier (F0
) to try the service.
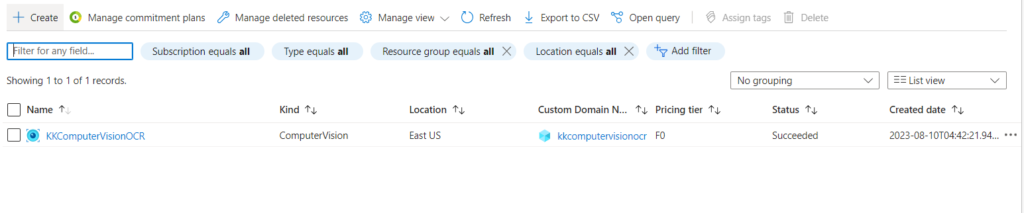
Once the resource is created successfully, you will be redirected to the available list.
Click on the name of the Computer Vision resource that you created just now.
Now, click on Keys and Endpoint.
This Key and Endpoint will be used in C# code to establish the connection with Azure AI computer Vision service.
Read printed and handwritten text using Azure Cognitive Service in .NET Core
Create a console application in Visual Studio 2022. Alternatively, you may use any version of Visual Studio.
First, add this package from the NuGet package manager – Microsoft.Azure.CognitiveServices.Vision.ComputerVision

Create a class called – ComputerVision.cs
Create a method called Authenticate which will return ComputerVisionClient type.
This method accepts 2 parameters first endpoint and the second parameter is the key, which we will copy from Azure Portal.
public ComputerVisionClient Authenticate(string endpoint, string key)
{
ComputerVisionClient client =
new ComputerVisionClient(new ApiKeyServiceClientCredentials(key))
{ Endpoint = endpoint };
return client;
}
Add a method to read text from the image file. This method accepts ComputerVisionClient
as the first parameter and file path or location as the second parameter.
public async Task ReadFileUrl(ComputerVisionClient client, string urlFile)
{
Console.WriteLine("Reading File");
Console.WriteLine();
// Read text from URL
var textHeaders = await client.ReadAsync(urlFile);
// After the request, get the operation location (operation ID)
string operationLocation = textHeaders.OperationLocation;
Thread.Sleep(2000);
// Retrieve the URI where the extracted text will be stored from the Operation-Location header.
// We only need the ID and not the full URL
const int numberOfCharsInOperationId = 36;
string operationId = operationLocation.Substring(operationLocation.Length - numberOfCharsInOperationId);
// Extract the text
ReadOperationResult results;
Console.WriteLine($"Extracting text from URL file {Path.GetFileName(urlFile)}...");
Console.WriteLine();
do
{
results = await client.GetReadResultAsync(Guid.Parse(operationId));
}
while ((results.Status == OperationStatusCodes.Running ||
results.Status == OperationStatusCodes.NotStarted));
// Display the found text.
Console.WriteLine();
var textUrlFileResults = results.AnalyzeResult.ReadResults;
foreach (ReadResult page in textUrlFileResults)
{
foreach (Line line in page.Lines)
{
Console.WriteLine(line.Text);
}
}
Console.WriteLine();
Console.ReadLine();
}
Currently, we have used an image path which is given by Microsoft and the image is shown below.
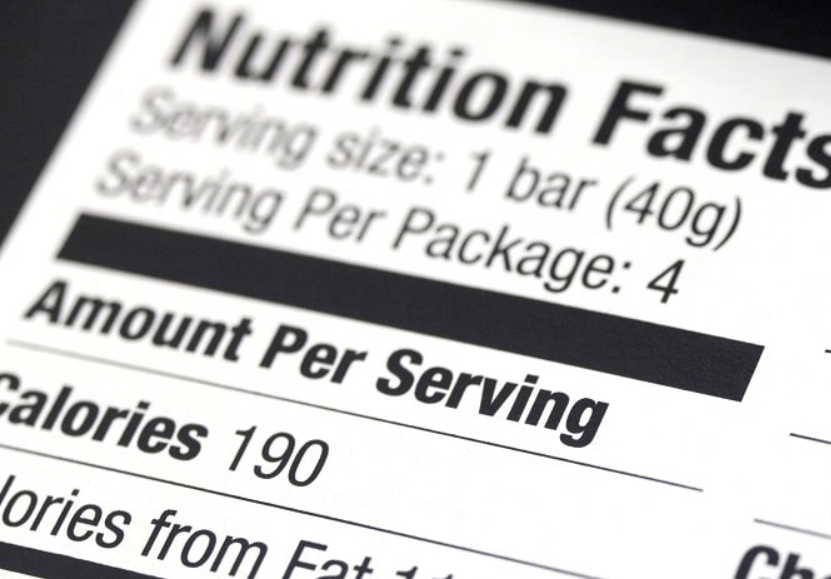
If you will run the application, you will get the below output.
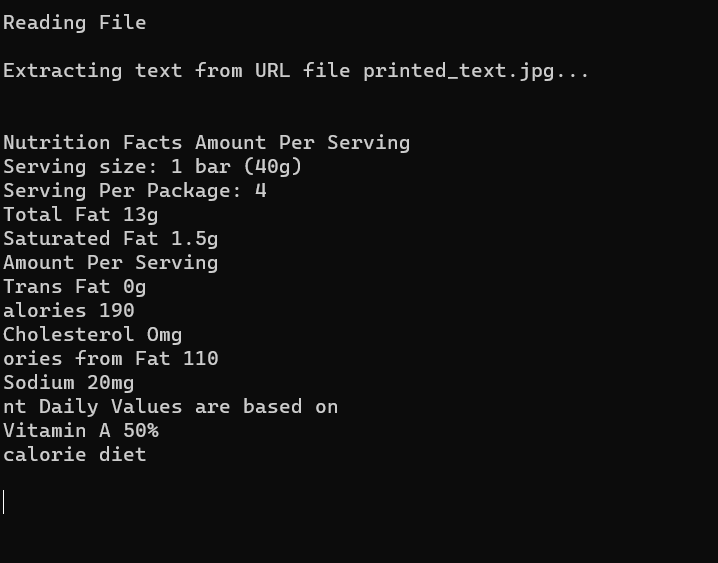
Instead of a URL, if you want to read text from a file located on your local disk, then you need to tweak your code.
//Comment this line
//var textHeaders = await client.ReadAsync(urlFile);
//And add below 2 lines of code to read file from local disk.
FileStream fileStream = new FileStream(urlFile, FileMode.Open, FileAccess.Read);
var textHeaders = await client.ReadInStreamAsync(fileStream);
Below is the output for hand-written text over plain white paper.
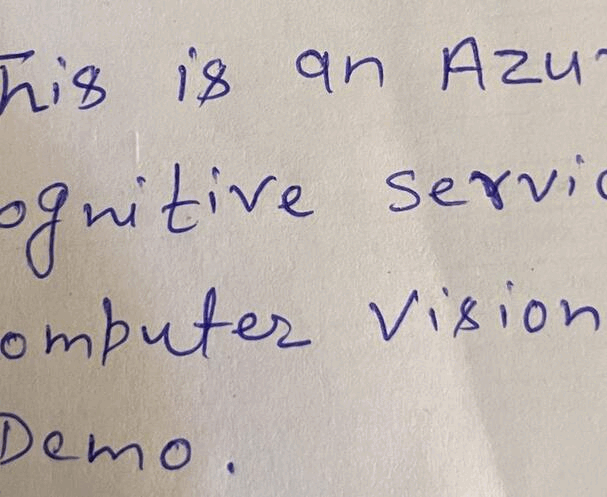
Takeaway
In this article, we learned about How to Extract Text from images using Azure Cognitive Services, Azure Cognitive Services reads text from Images with handwritten text. To demonstrate this functionality, we created an Azure AI Computer Vision service in Azure Portal and consumed this service in C#. NET Core console application.
We learned how to read a file from a public URL and convert it into text, we also converted an image stored on a local disk to text form.
Hope you have enjoyed this article!!!
Please do share the article within your tech group and write your comment below, in case of any feedback.
Happy Learning đŸ™‚