In this article, we are going to learn about Data Protection in ASP.NET Core: what it is, why we need it and how to implement it in .NET Core-based applications.
What is Data Protection?
Let’s first understand the concept of data protection. It is a mechanism to apply security level to any data from unauthorized access. Data Protection plays a very vital role especially when we move data from one channel to another. For example, – ASP.NET Core API, where we send and receive data from various sources or expose sensitive information in URLs.
So, whenever we transfer data over the network, we need to protect it under Data Protection.
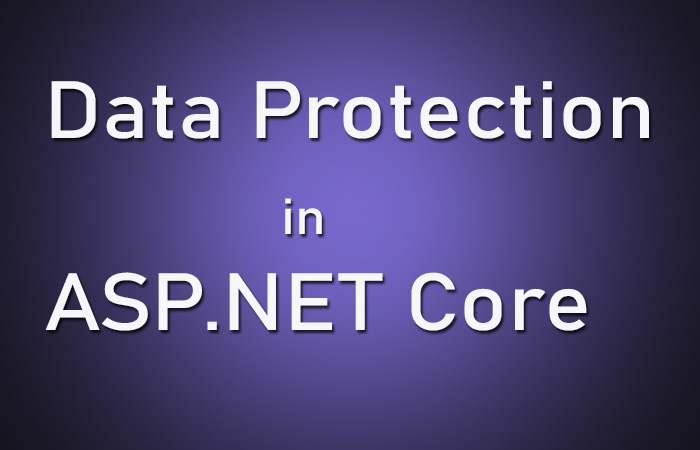
Why do we need Data Protection?
Data protection is crucial, as it protects the data from unauthorized access. When we exchange data through API, data is risky to be hacked or tampered with. To avoid this, we use data protection.
In this mechanism, we encrypt the data so that no one can temper or change the data in the middle of the network.
Data Protection in ASP.NET Core
Data Protection in ASP.NET Core provides cryptography-based API to protect data. And, Microsoft uses Microsoft.AspNetCore.DataProtection
namespace to implement data protection.
The namespace Microsoft.AspNetCore.DataProtection
is the core of data protection in ASP.NET Core. It contains cryptographic methods, configuration and key management and contains the below interfaces-
- IDataProtector – It is an interface which provides services to encrypt and decrypt data.
- IDataProtectionProvider
How to implement Data Protection in ASP.NET Core-based application?
In this section of the article, we will see a code snippet to implement data protection in the ASP.NET Core project.
Firstly, let’s create a Web API project in Visual Studio 2019 or 2022.
Secondly, add the below line within the ConfigureServices()
method in the startup.cs
file to register in IServiceCollection
.
services.AddDataProtection();
Now, let’s create a data protector object with IDataProtector.
private readonly IDataProtector _dataProtector;
Add the IDataProtectionProvider
type parameter to the constructor of the controller class. For this demonstration, we are using a TestController
class, so the code snippet will be:
public TestController(IDataProtectionProvider dataProtectionProvider)
{
_dataProtector = dataProtectionProvider.CreateProtector("Secret Key");
}
Finally, we will use a dataprotector instance to protect the data.
public string GetMessage()
{
string message = "This is a secret message to team";
return _dataProtector.Protect(message);
}
Let’s see the complete code snippet:
namespace DataProtectorDemo.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class TestController : ControllerBase
{
private readonly IDataProtector _dataProtector;
public TestController(IDataProtectionProvider dataProtectionProvider)
{
_dataProtector = dataProtectionProvider.CreateProtector("Secret Key");
}
[Route("GetMessage")]
public string GetMessage()
{
string message = "This is a secret message to team";
return _dataProtector.Protect(message);
}
}
}
Let’s see the output –
Run the application and hit the API either from the browser or from Swagger or POSTMAN.
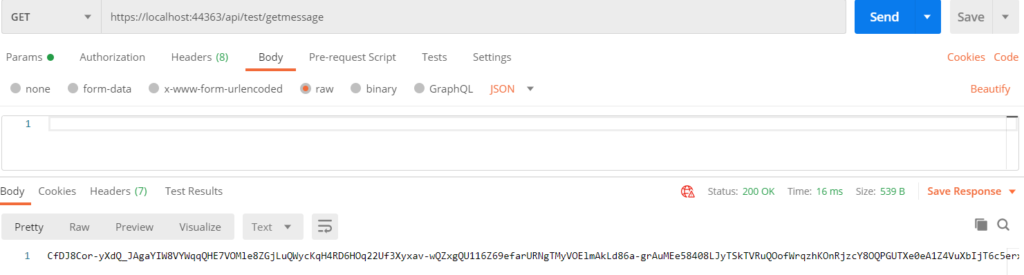
Here, we see the data in a protected way.
Let’s verify the result by unprotecting the same data.
Change the Action method like the code snippet below:
[Route("GetMessage")]
public IActionResult GetMessage()
{
string originalMessage = "This is a secret message to team";
string protectedMessage = _dataProtector.Protect(originalMessage);
string unProtectedMessage = _dataProtector.Unprotect(protectedMessage);
return Ok(new { originalMessage, protectedMessage, unProtectedMessage });
}
Protect() method
This method accepts plain text and then protects the plain text cryptographically.
Unprotect() method
This method accepts protected data as a parameter and then unprotects the data cryptographically. In other words, It converts the encrypted data to plain text.
In Postman, we will see the below output.
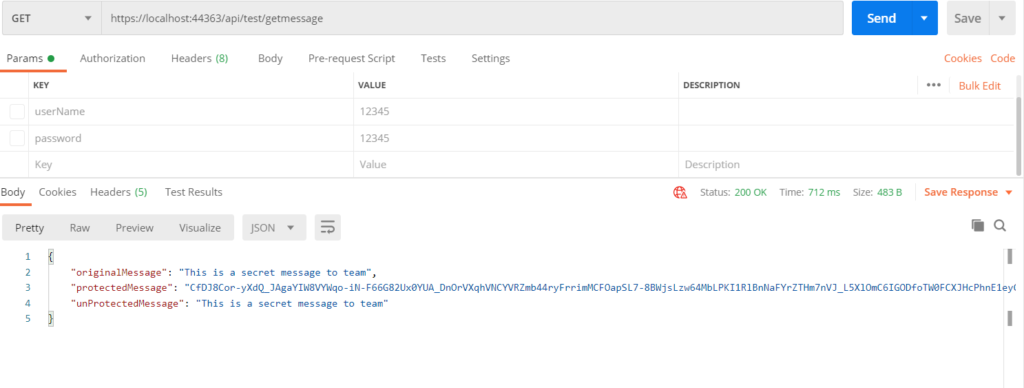
Here, We are first displaying the original message then protecting the message and finally unprotecting the protected data.
Takeaway
We have gone through the implementation of data protection in ASP.NET Core. We know the significance of data protection and how this is useful in protecting sensitive information. We also learnt the way to implement data protection in ASP.NET Core.
To summarize, we came through below headlines –
- What is Data Protection?
- How to implement Data Protection in ASP.NET Core?
- We can encrypt sensitive information in URLs and also in API responses.
Hope you enjoy this article.
For more details, please read this from Microsoft: Get started with the Data Protection APIs in ASP.NET Core