C# programming offers numerous iteration statements to iterate through an array. Some of the iteration statements are for loop, foreach loop. In this article, we will learn the use of the foreach loop in C#.
Understand the Iteration concept
Iteration is the execution process for a defined number of times until the given condition is true. There are 4 ways of iteration mechanism in C#:
- for loop
- while loop
- do-while loop
- foreach loop
In this article, we will explore the foreach loop in detail.
Foreach Loop Concept and Syntax in C#
C# foreach loop is an iteration mechanism to loop through all elements in an array. So, the foreach
loop keeps iterating until the items or elements are available in the given collection.
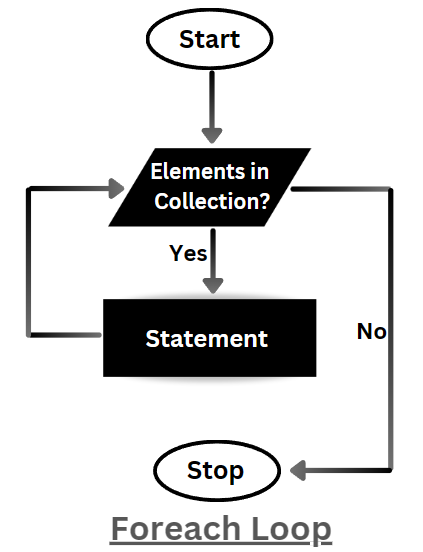
Let’s see the syntax to write the foreach
loop in C#:
foreach (int value in numbers)
{
Console.WriteLine(value);
}
Here, int
is the data type, value
the looping variable and numbers
is the collection.
Parallel Foreach Loop
In C#, Parallel.ForEach()
provides an iteration mechanism to iterate through a collection similar to the foreach
loop, but it does the same in a parallel way.
In general, foreach
loop executes the process sequentially. It means the loop runs one by one from a single thread.
On the other side, the Parallel.ForEach()
loop runs parallelly and uses multiple threads. Parallel.ForEach()
makes the task faster and it was introduced in .NET 4.0.
Let’s look at the syntax of Parallel.ForEach()
loop in C#:
Parallel.ForEach<TSource>(IEnumerable<TSource> source, Action<TSource> body)
Let’s see Parallel.ForEach()
loop with the help of an example:
List<string> product = new List<string>();
product.Add("Laptop");
product.Add("Mouse");
product.Add("TV");
product.Add("Bike");
Parallel.ForEach(product, product =>
{
Console.WriteLine("Product Name: {0}", product);
});
In the code snippet, we have a list of strings and we are printing each item of the collection using the Parallel.ForEach()
loop.
Iterate an Array using Foreach Loop in C#
So, we will iterate through an array using the foreach
loop.
To demonstrate this, we will use an array.
Let’s look at the code snippet:
string[] cars = { "BMW", "Honda", "Ford", "Toyota" };
foreach (string car in cars)
{
Console.WriteLine(car);
}
Here, we are declaring cars array or string. Then we print the item of the array using a foreach
loop.
C# Foreach Loop with List Collection
Now, we will use the foreach
loop to iterate through a list collection.
Let’s look at the code snippet below:
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
In the code snippet, we have a List<int>
of integers. We are then using a foreach
loop to iterate through it.
Limitations of Foreach Loop
There are a few limitations with the foreach loop. These are:
- They don’t have an index value to track an item.
- The foreach loop can traverse only forward. It can not move in the backward direction.
Parallel foreach vs foreach performance C#
Parallel.ForEach
loop, as the name suggests, runs the process in multiple threads parallel. On the other hand, a foreach
loop runs on a single thread and executes one by one. To use Parallel.ForEach
we need the System.Threading.Tasks
namespace.
For bulk and huge data, we can go with Parallel.ForEach
loop and for simple iterations with fewer data foreach
is a good choice.
For Loop Vs Foreach Loop
Let’s understand the differences between for
and foreach
loop in C#.
With for loop, we have minimum and maximum boundaries to start and stop the loop. However, the foreach loop does not have any defined boundary. The foreach
loop iterate until the elements or items are available in the collection.
For loop can iterate in backward and forward directions. The foreach loop can traverse in the forward direction only.
Let’s look at the for
loop syntax:
for (Statement 1; Statement 2; Statement 3)
{
// code block to be executed
}
Here, we are using a for loop. Statement 1 is the start point or initial boundary, Statement 2 is the maximum limit or boundary and Statement 3 is the counter increment and decrement.
Conclusion
So, in this article, we explored the foreach loop and parallel foreach loop. We also compare the for and foreach loop in C#.
Keep following: SharePointcafe.NET