In this article, we will explore Variables and Data Types in C#. Before we proceed further, let us first understand what Data is. This article is helpful for beginners or preparing for an Interview as a C# programmer.
What is Data?
In computer programming, data is one of the most essential parts of any application or project. A computer stores the data in a series of bits.
Q. What is a bit?
Answer: A bit is a binary representation of the data in the form of 0 and 1.
The single bit doesn’t make any sense but 8 bits in a sequence makes a byte. So, 1 byte = 8 bits.
What is a Variable in C#?
A variable is a name stored in a memory location and we must declare a variable before we use it. There are multiple types of variables available in C#.
- Local Variables
- Instance Variables
- Static Variables
- Constant Variables
- Readonly Variables
What are Data Types in C#?
The data type in C# defines how much memory we need for our variable. There are numerous data types available in C# for various purposes. Some of the data types are int
, string
and bool
.
.NET class library defines each data type in C#. For example, int
data type is defined in System.Int32
library.
Value Type and Reference Type
If you are a beginner, you will think about what is Value Type and Reference Type, and what is the relation to data type in C#.
In simple words, Value type and Reference type are the ways to store a variable in memory.
A reference type variable stores the reference to their data or we can say they point to the value which is stored at some other location. But, in value type, they directly contain the data.
Some of the examples of value type are int
, string
, bool
, float
Example of Reference data type: String
, Class
, Interface
Variable Declaration in C#
Let’s see the way we declare variables in C# language.
int number = 10;
string countryName = "India";
float amount = 10.2f;
char name = 'a';
bool isSelected = true;
Here, we are declaring multiple variables of different data types. You may notice that character f is there while declaring float value.
In C# language, there are data types which need a suffix once we declare the variable. These data types are – unsigned integers
, float
, long
, double
, and decimal
.
uint value1 = 100u; // Unisigned integers
float floatValue = 10.5f; // Float
long longValue = 51355452121310l; // Long
double doubleValue = 2156234.211d; // Double
decimal decimalValue = 550.20m; // Decimal
Range of Data Type
There are several data types available in C#. So, you may think why so many data types?
Every data type in C# has its limitations and range. For example – A byte data type can range from 0 to 255, and a bool
data type may accept only two values true or false.
Data Type | Range |
byte | 0 to 255 |
short | -32,768 to 32,767 |
int | -2,147,483,648 to 2,147,483,647 |
long | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | -3.402823e38 to 3.402823e38 |
decimal | (+ or -)1.0 x 10e-28 to 7.9 x 10e28 |
double | -1.79769313486232e308 to 1.79769313486232e308 |
bool | true or false |
string | A sequence of Unicode characters |
DateTime | 0:00:00am 1/1/01 to 11:59:59pm 12/31/9999 |
Default Values
When we assign a value to a variable it stores that data, but how to assign a default value?
Every data type in C# has its default value, for example, an integer’s default value is 0 (zero). But we can assign a default value to all data types in a similar way.
Let’s look into the code which assigns a default value.
int a = default;
bool b = default;
char c = default;
decimal d = default;
float e = default;
Console.WriteLine("integare Default Value: " + a);
Console.WriteLine("boolean Default Value: " + b);
Console.WriteLine("char Default Value: " + c);
Console.WriteLine("decimal Default Value: " + d);
Console.WriteLine("float Default Value: " + e);
Console.ReadLine();
Here, we are using a keyword default
to assign the value. Press F5 and see the output.
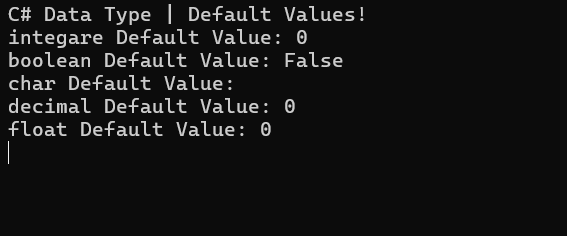
Data Conversion in C#
In C# language, we can convert one data type to another using implicit or explicit conversion. In this section of the article, we will see Implicit and Explicit conversion.
Implicit Type Data conversion in C#
C# compiler automatically converts smaller-size data types to larger data types. For example – Conversion from int
to double
data type.
int number = 100;
double val = number;
Here, we declare a variable number as int
type. In the next line, we are assigning the int
value to a variable of double
type. So, this is a valid conversion and will compile successfully.
Explicit Data Conversion in C#
A C# compiler applies explicit data conversion when we request a conversion explicitly from one data type to another. To do this, we use cast expression.
double amount = 5123.50;
int number = (int)amount;
Here, we are declaring a variable of double
type and then we assign the double
value to int
variable. This code will compile with no error because we are doing explicit conversion using (int)
which is a cast expression.
But, what will happen when we do not use cast expression? This will throw a compile time error as –
Cannot implicitly convert type ‘double
‘ to ‘int
‘. An explicit conversion exists (are you missing a cast?)
So, in this way, we can convert a int
type to string
. But, conversion from string
to int
is not possible unless the numerical value input as string
.
Conclusion
In this article, we explored Variables and Data Types in C#. We are now comfortable with Value Type and Reference Type. We can also apply data conversion using C# code.
Write more code snippets and try to convert data type randomly and see the error details. This will give a wider perspective on data conversion in C#. Note that Variables and Data Types in C# are one of the most important topics from an interview point.
Hope, you like this article. Please do share this within your social media group and please give your feedback in the comment box below.
Keep Following – SharePointCafe.Net