In this article, we will understand the Factory Design Pattern with C# code.
It is one of the most popular design patterns used in the programming world. There are mainly 3 types of design patterns which are sub-divided into multiple patterns.
Factory Design Pattern comes under Creational Pattern and the other 2 are Structural Patterns and Behavioral Patterns.
For this article, we will stick to the Factory Design Pattern and its implementation with C# code. You may also watch a video available at the bottom of the page which demonstrates this design pattern in C#.
What is a Design Pattern?
In the programming world, a design pattern is an overall solution for a usually happening issue in application design and architecture. A good design pattern makes your code clean and maintainable.
A design Pattern is a blueprint or template to define the solution for a problem.
Factory Design Pattern Implementation with C# code
In Factory Design Pattern, the client doesn’t know about the actual classes and their details. Instead of instantiating or creating objects of actual class, we use an interface for creating objects.
We use a mediator class for initializing the object of Interface and then in the client program, we use the method of mediator class which then can call the methods of actual classes.
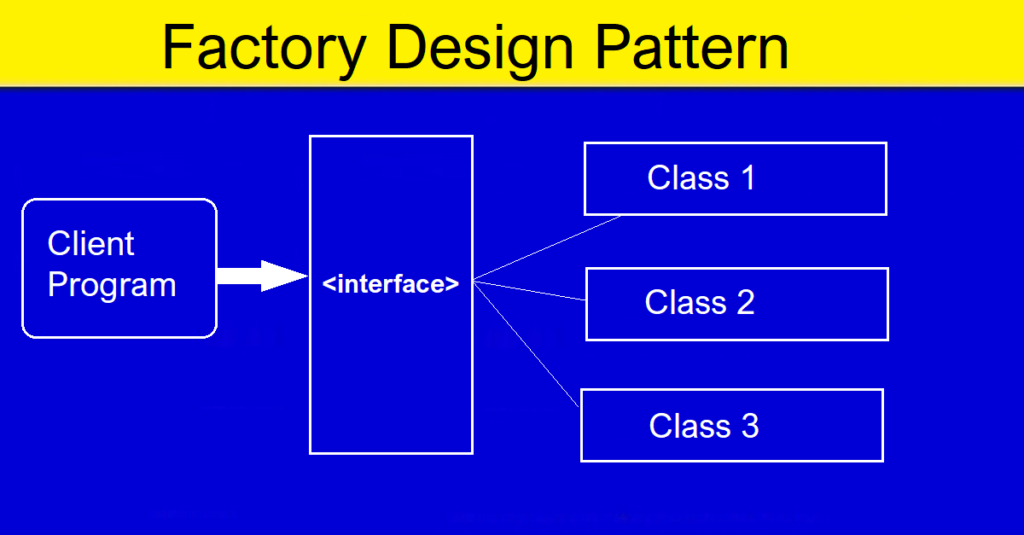
Let’s see the Factory Pattern with C# code step by step.
- Create an Interface and name it
IFactoryPatternDemo
interface IFactoryPatternDemo
{
string GetDetails(int id);
}
2. Create a class Employee.cs
and inherit it from IFactoryPatternDemo
class Employee : IFactoryPatternDemo
{
public string GetDetails(int EmpId)
{
return "Employee Name is - Tom";
}
}
3. Create another class Product.cs and inherit it with the same interface.
class Product : IFactoryPatternDemo
{
public string GetDetails(int Pid)
{
return "Product Name is - Laptop";
}
}
4. Create one more class FactoryPatternDemo.cs
. This is our mediator class.
class FactoryPatternDemo
{
public static IFactoryPatternDemo FactoryObject(string objName)
{
IFactoryPatternDemo factoryPatternDemo = null;
if (objName == "emp")
{
factoryPatternDemo = new Employee();
}
else
{
factoryPatternDemo = new Product();
}
return factoryPatternDemo;
}
}
5. Now, we will call the methods of Employee class and Product class. But instead of instantiating these 2 classes, we will use FactoryPatternDemo
.
static void Main(string[] args)
{
string objectName = "emp";
IFactoryPatternDemo factoryPatternDemo = FactoryPatternDemo.FactoryObject(objectName);
Console.WriteLine(factoryPatternDemo.GetDetails(1));
}
In the above code, you can change the value of the objectName variable to either “emp” or “product”. Accordingly, the client can call the method(s) for the respective class.
Below is the complete code-
I have used all the classes and one interface in a single file. You may create separate files at your convenience.
using System;
namespace DesignPatterDemo
{
class Program
{
static void Main(string[] args)
{
string objectName = "emp";
IFactoryPatternDemo factoryPatternDemo = FactoryPatternDemo.FactoryObject(objectName);
Console.WriteLine(factoryPatternDemo.GetDetails(1));
}
}
class FactoryPatternDemo
{
public static IFactoryPatternDemo FactoryObject(string objName)
{
IFactoryPatternDemo factoryPatternDemo = null;
if (objName == "emp")
{
factoryPatternDemo = new Employee();
}
else
{
factoryPatternDemo = new Product();
}
return factoryPatternDemo;
}
}
interface IFactoryPatternDemo
{
string GetDetails(int id);
}
class Employee : IFactoryPatternDemo
{
public string GetDetails(int EmpId)
{
return "Employee Name is - Tom";
}
}
class Product : IFactoryPatternDemo
{
public string GetDetails(int Pid)
{
return "Product Name is - Laptop";
}
}
}
So, in this way instead of exposing actual classes to clients, we have used an intermediate class and interface to deal with it.
Hope you are now clear with the Factory Design Pattern methodology and its concept.
If you have any feedback, please write it down in the comment box below.
Please share this article among your tech group and please do like our Facebook page to join us and get the latest updates.
You may subscribe to the YouTube channel for the latest videos on Programming, Azure and .NET Core Technologies.