A data structure is one of the most important parts of any programming language. In this article, we will see Data structure and its types in detail.
What is Data Structure?
It is a methodology of organizing data in a specialized format on a computer so that the information can be organized, processed, stored, and retrieved quickly and effectively. They are a means of handling information, rendering the data for easy use.
A data structure is not only used for organizing the data. It is also used for processing, retrieving, and storing data.
In computer science and computer programming, a data structure might be chosen or intended to store data to utilize it with different algorithms.
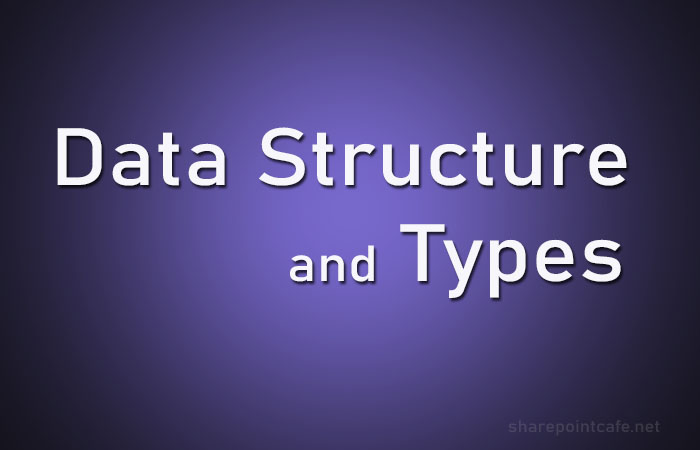
Data structures are the structure blocks for modern software applications. They are planned by forming data components into a sensible unit.
It isn’t simply essential to implement data structures into your application, it is additionally critical to pick the right data structure type for the right data. So, let’s explore the types of data structures.
Types of Data Structure
There are 2 main types of Data Structure.
- Linear Data Structure – Data elements in a linear data structure are linked to one another in a sequential arrangement, with each element linked to the elements in front of and behind it.
- Non-Linear Data Structure – Data structures where data elements are not placed sequentially or linearly are called non-linear data structures.
Types of Linear Data Structure
Array
It is the collection of similar data types. An array can be defined as an ordered collection of items indexed by contiguous integers.
Arrays are used in almost all programming languages.
There are 2 types of Array –
- One Dimensional Array
- Multi-Dimensional Array
Array Syntax in C#
< DataType > [] < Array Name >
Example –
int[] data;
string[] student;
Queue
A queue is another linear data structure and it follows the FIFO order.
FIFO stands for First-In-First-Out, so the element inserted first is removed first.
FIFO Principle –
A Queue is like a line waiting at booking windows, where the first user in line is the first to be served.
Operations in Queue Data Structure
Below are the operations that can be performed on Queue.
- enqueue – Operation to add or insert the new item.
- dequeue – Process to remove an item from Queue.
Stack
A stack is a linear data structure which follows a particular order in which the operations are performed.
It follows LIFO or FILO principle.
LIFO stands for Last-In-First-Out. FILO stands for First-In Last-Out.
Operations in Stack Data Structure
Below are the operations that can be performed on Stack.
- push() – This operation allows to addition or insertion of new items into the stack.
- pop() – It allows to removal or deletes items from the stack.
Linked List
It is a Linear data structure where elements are linked with the help of pointers like the below image –
Linked List has nodes which contain data and reference to the next adjacent node.
Types of Linked Lists
- Single Linked List – An element can traverse in Only one direction.
- Double Linked List – An element can traverse in both directions either Forward or Backward.
- Circular Linked List – In this type of Linked List, the last node of the list contains the reference of the first node.
Basic Operation on Linked List
- Traversal – Process to traverse from one node to another.
- Insertion – To insert a new node in the List.
- Deletion – To remove or delete a node.
- Search – Allows searching an element.
- Sort – To arrange the Linked List in the given order.
- Merge – Allows to merge 2 Linked Lists into one.
Types of Non-Linear Data Structure
Tree
A tree is a non-linear data structure that represents the hierarchy. It is a set of nodes that are linked together to form a hierarchical structure.
A tree may have a finite set of one or more nodes. One special node in the Tree is called the Root Node.
Components of Tree Data Structure
- Root Node
- Child Node
- Edge
- Siblings
- Leaf Node
- Height of Tree
- Degree of Node
Graph
A Graph is a non-linear type of data structure with vertices and edges. The vertices are also referred to as nodes and the edges are lines or arcs that connect any two nodes in the graph.
Types of Graph Data Structure
- NULL Graph
- Trivial Graph
- Undirected Graph
- Directed Graph
- Connected Graph
- Disconnected Graph
- Regular Graph
- Cycle Graph
Data Structure Operations
Below are the major operations that can be performed on any type of Data Structure.
- Searching
- Sorting
- Insertion
- Deletion
Benefits of using Data Structure
Data structure became very useful for a complex type of application due to below points –
- It provides abstraction and reusability.
- Using the right Data Structure type helps developer to save time while performing operations on large and complex datasets.
- If you choose the right Data Structure, then it is easier to manipulate a huge amount of data.
Important Data Structure Interview Questions
Based on the above content, a candidate will be able to answer the below questions in an interview.
- Explain types of Data Structure.
- What is a Stack?
- What is FIFO Principle?
- One-Dimensional array vs Two-Dimensional array
- What is a Linked List?
- What is Queue?
- Explain Tree Data Structure.
- What are types of Graph Data Structure?
Takeaway
Data Structure is a mechanism for managing data quickly and efficiently.
This article mainly focuses on Data Structure and its types. This is useful for fresher-level candidates who are preparing for an interview. It is applicable to all programming languages background.
In upcoming articles, I will write more about Data Structure and Algorithms. Keep Following – SharePointCafe.NET